Chapter I – Computer Programming, The purpose of all computers
In this chapter you will learn:
1) The purpose of all computers
- Why computers are a lot like a game of Monopoly. (What computer and Board Games have in common.)
- What an algorithm is and how your computer follows them
- How to write your own computer algorithms
A computer is a machine that follows a step by step list of instructions. Another name for a step by step list of instructions is an algorithm. Examples of algorithms people encounter on a day to day basis are a list of items to buy at a grocery store, the instructions to assemble a bicycle, and the rules that everyone is supposed to follow when they come to a traffic light. The purpose of all computers, the reason for their existence, is to follow algorithms. Another name for the algorithm a computer follows is a computer program.
In a sense, a computer follows the instructions in an algorithm in exactly the same way that a person follows the rules(instructions) in a game of Monopoly. In Monopoly, there are a sequence of squares on a board. Each square holds an instruction. When a player lands on a square, the player reads the instruction in the square and then performs this instruction (Go to Jail, Collect $200.00 when you pass GO, etc.)
Literally, this is the way a computer follows an algorithm:
1) The monopoly squares are analogous to computer memory.
2) The instructions in each monopoly square are analogous to algorithm instructions stored in the computer memory. Each monopoly square holds one monopoly game instruction. Each computer memory “square” holds one computer algorithm instruction.
3) The player reads the instruction on the square they land on and performs that instruction. The computer reads the instruction on the memory “square” it “lands on” and performs that instruction.
4) The official parker brothers game of monopoly comes with a set of exactly 128 rules and 40 Squares. The computer you will read about in this book has only 8 instructions and 64 memory squares. By comparison, a commercial computer, such as the Intel Pentium 4 Processor, contains hundreds of instructions and millions of squares.
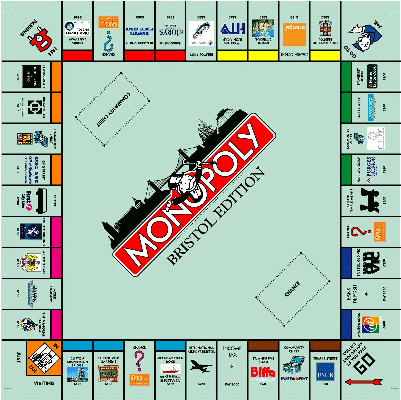
Monopoly Board with 40 “memory squares”. Each square contains an instruction such as “Go to jail” or “collect $200.00”
The digital computer in this book with 64 “memory squares”. Each square can contain one of 8 instructions such as “Go to a new memory square” or “add the number stored in square 15”
The game of monopoly comes with its instructions FIXED into place on the squares of the monopoly board. However, what makes a computer useful, in fact, what makes this computer and ALL computers “programmable”, is that you can place any of these 8 instructions you like in any place on the board (in any memory square) you want.
In the remainder of this book, I will describe the design and operation of a simple digital computer that I call the SIMCO, short for simple computer. This chapter will present the operation of the SIMCO as the rules of a board game, the game of “SIMCO”. By learning the rules of this game and following along with the examples, you will learn how to program the SIMCO computer and gain an overview of how the SIMCO follows the programming instructions you place in its memory squares.
Learning to Program…Learning the RULES to the game of SIMCO.
The game of SIMCO is played on a board with 64 memory squares, numbered 0 to 63. In the game of SIMCO, each square’s number is also called an “Address”. Play begins at square 0 and proceeds according to the following 6 rules:
- Start at square 0.
- Read the instruction found in the square you are on.
- Perform the instruction.
- Jump to the next sqaure and repeat these rules, beginning with rule 2.
- If a square does not contain an instruction, simply jump to the next square and repeat these rules, beginning with rule 2.
- After you have landed on sqaure 63, the next square to jump to will be square 0. Repeat these instructions beginning with rule 2.
In addition to the 64 memory squares, the SIMCO also has 5 special “scratch pad” squares called Registers. For now, only 3 of these 5 squares are important. Here is a list of these 3 squares and what they do:
- Accumulator Register: Holds the result of an Add, Subtract, or Multiply instruction
- Memory Address Register: Keeps track of which square the computer is currently on
- Location Counter Register: Keeps track of which square the computer will be jumping to.
- Instruction Register: Keeps track of which algorithm instruction the computer is to perform
The instruction register holds the number of the instruction found in the memory square the computer is currently on.
There are 8 instructions that can be placed onto the SIMCO’s memory squares, numbered 0 to 7. These instructions are listed in order below:
- Add 2 numbers together and store the result in the accumulator square.
For example, the instruction ADD 5 will add the number found in memory square 5 to the value in the accumulator, and then write the sum of these 2 numbers back to the accumulator, overwriting the old value in the accumulator.
- Subtract one number from another number and store the result in the accumulator square.
For example, the instruction SUB 5 will subtract the number found in memory square 5 from the value in the accumulator, and then write the difference of these 2 numbers back to the accumulator, overwriting the old value in the accumulator.
- Multiply a number by 2
For example, if you land on a memory square containing the instruction MUL 17, the number in memory square 17 is multiplied by 2 and the result is written to the accumulator square, overwriting whatever number was previously in the accumulator.
- “Jump” to a new square
For example, assuming you land on memory square 17 containing the instruction JMP 8, You must move immediately to memory square 8, skipping over all of the squares in between.
- “Jump” to a new square ONLY if the accumulator square contains the number 0.
For example, if you land on a memory square containing the instruction JZ 45 and the value of the accumulator square is 0, you must immediately move to memory square 45, skipping over all of the square in between. If the number in the accumulator square is not 0, then you simply proceed to the next square as according to rule 4 above.
- Write the number zero to the accumulator.
For example, if you land on a memory square containing the instruction CLA, the number 0 gets written to the accumulator square, overwriting whatever number was previously in the accumulator.
A computer program to add the numbers 32 and 56 together
Below is a picture of memory squares 0 to 7 of the 64 memory squares. In memory squares 0 to 4 are instructions. By following the 6 rules of the game of SIMCO above and performing the instructions found in these memory squares, the computer will add the numbers found in memory squares 5 and 6 together and write the sum in memory square 7. The instructions found in memory squares 0 to 4 are called an algorithm, or computer program.
Note:
1 The left side of the picture below shows the 5 special register squares mentioned above.
2 The right side of the picture below shows memory squares 0 to 7 of the 64 memory squares.
3 The number to the left of each memory square identifies that square. It is the square’s “address”.
4 The number highlited in yellow is the memory square the computer is currently on.
5 Depicted in each memory squares 0 to 4 are instructions. For instance, memory square four contains the instruction STP, which means “stop the program”.
Here is how the program works, step by step:
Rule 1: Begin at Square 0
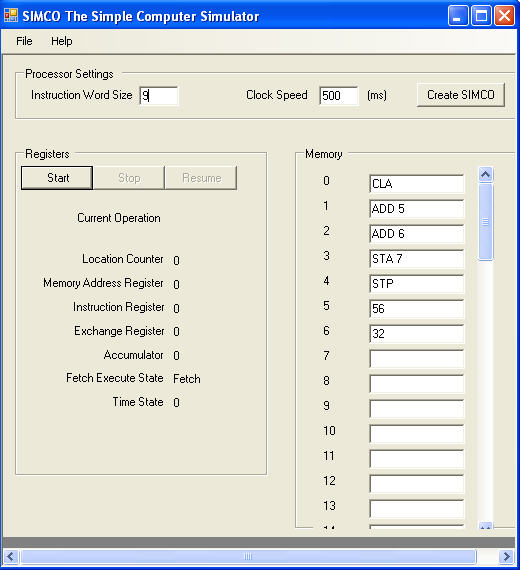
Rules 2 and 3) Read the instruction found in the square you are on and Perform the instruction.
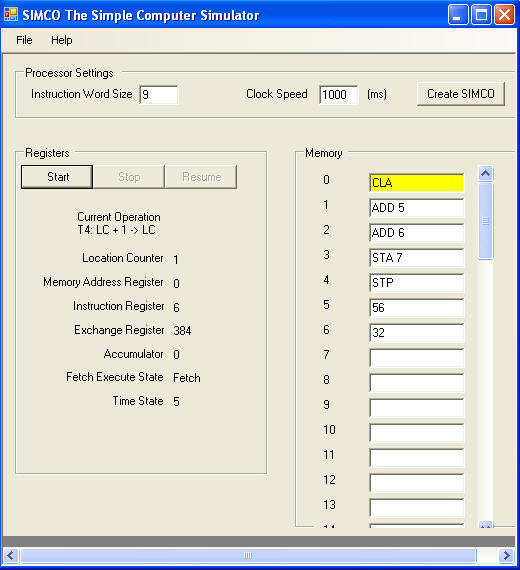
Rule 2: Read the instruction in the memory square you are on into the instruction Register. Memory square 0 has instruction CLA(“Clear the Accumulator”). CLA is instruction number 6. This instruction number is placed into the instruction register so that the SIMCO knows what instruction it is to perform. In the picture above you see that the number 6, representing instruction CLA, is now in the instruction register.
Rule 3: Perform the instruction. Instruction CLA puts the number 0 into the Accumulator Register.
The Location counter register holds the number of the next square to jump to. We are currently on memory square 0, so the next memory square to jump to is square number 1.
Rule 4 Jump to the next square and repeat these rules, beginning with rule 2.
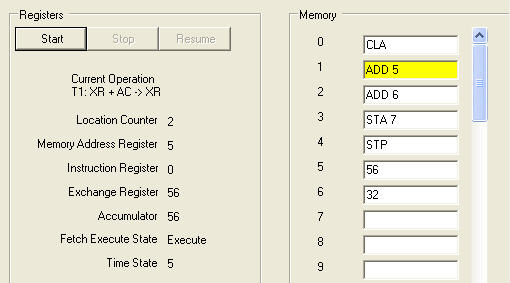
Rule 4: The SIMCO was on square 0, so now it jumps to the next sqaure, square 1. The Location counter ALWAYS holds the value of the next square to jump to.
Rule 2: Read the instruction in the memory square you are on and place it into the instruction Register. Memory square 1 has instruction ADD 5. ADD is instruction number 0, so 0 is placed into the instruction register. This instruction tells the SIMCO to add the value at memory square 5 to the value in the Accumulator.
Rule 3: Perform the instruction. The Accumulator had the value 0 in it. Square number
5 has the value 56 in it. 0 + 56 = 56. This sum, 56, is written to the Accumulator, as depicted above.
Rule 4: Jump to the next sqaure and repeat these rules, beginning with rule 2.
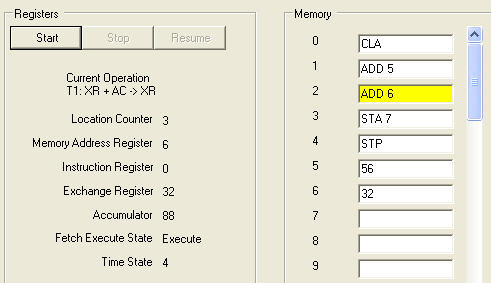
Rule 4: The SIMCO was on square 1, so now it jumps to the next sqaure, square 2. The Location counter is incremented to hold the value of the next square to jump to.
Rule 2: Read the instruction in the memory square you are on into the instruction Register. Memory square 2 has instruction ADD 6. ADD is instruction number 0, so this is written to the instruction register. This instruction tells the SIMCO to add the value at memory square 6 to the value in the Accumulator.
Rule 3: Perform the instruction. The Accumulator had the value 56 in it. Square number
6 has the value 32 in it. 56 + 32 = 88. This sum, 88, is written to the Accumulator.
Rule 4: Jump to the next sqaure and repeat these rules, beginning with rule 2.
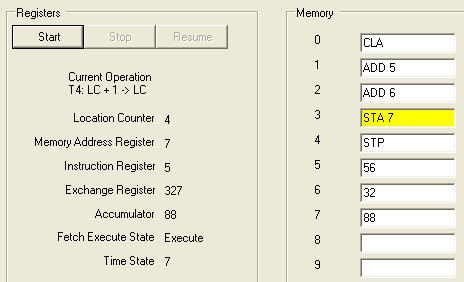
Rule 4) The SIMCO was on square 2, so now it jumps to the next sqaure, square 3. The Location counter is incremented to hold the value of the next square to jump to, which will be square 4.
Rule 2) Read the instruction in the memory square you are on into the instruction Register. Memory square 3 has instruction STA. STA is instruction number 5, so 5 is written to the instruction register. This instruction tells the SIMCO to STore (Write) the value in the Accumulator to memory square 7.
Rule 3)Perform the instruction. The Accumulator has the value 88 in it. This value is written to memory square 7, as shown in the picture above.
Rule 4) Jump to the next sqaure and repeat these rules, beginning with rule 2.
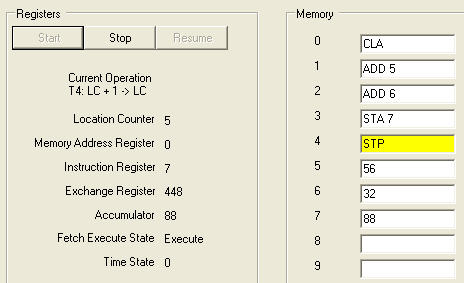
Rule 4) The SIMCO was on square 3, so now it jumps to the next sqaure, square 4. The Location counter is incremented to hold the value of the next square to jump to, which will be square 5.
Rule 2) Read the instruction in the memory square you are on into the instruction Register. Memory square 4 has instruction STP. This instruction tells the SIMCO to STOP following the algorithm. When this instruction is performed, the SIMCO will
No longer jump to new memory squares and perform the instructions in them.
Rule 3)Perform the instruction. The SIMCO stops following the program, and memory
Location 7 now has the value 88 in it, which is the sum of 32 and 56.
Here is a listing of the Computer program we just stepped through, line by line:
A program to add the numbers 32 and 56 together
0) CLA ; place the number 0 in the accumulator square
1) ADD 5 ;Add the value found at memory square 5 to the accumulator -- this value is 56
2) ADD 6 ;Add the value found at memory square 6 to the accumulator -- this value is 32
3) STA 7 ;write the value in the accumulator to memory square 7
4) STP ;stop the program
5) 56
6) 32
7) ;the sum of 32 and 56 will be written here
Below are other simple programs that can be run on the SIMCO. These short programs will help familarize you with the SIMCO's 8 programming instructions
A program to subtract one number from another
;This program subtracts 32 from 56 and places the result in memory location 7
0 CLA ;the instruction to put a zero in the accumulator is in
1 ADD 4 ;Add the value found at memory square 3 to the accumulator -- this value is 32
2 SUB 5 ;Subtract the value found at memory square 4 from the accumulator
3 STA 7 ;write the value in the accumulator to memory location 7
4 56
5 32
6 STP ;stop the program
7 ;56 - 32 will be written here
A Program to subtract one number from another, generating a negative number
;A program to subtract 56 from 32, generating a negative number
0 CLA ;the instruction to put a zero in the accumulator is in
1 ADD 4 ;Add the value found at memory square 3 to the accumulator -- this value is 32
2 SUB 5 ;Subtract the value found at memory square 4 from the accumulator
3 STA 7 ;write the value in the accumulator to memory location 7
4 32
5 56
6 STP ;stop the program
7 ;the sum of 32 and 56 will be written here
An “Endless loop” program to continuosly ADD the number 1 to the accumulator, then writes the value in the accumulator to memory square 5
;A program to endlessly add the number 1 to itself.
0 CLA ;clear the accumulator
1 ADD 3 ;Add the value found at memory square 3 to the accumulator
2 STA 5
3 3; the value 1 is stored at memory address 3
4 JMP 1 ;jump back to memory square 1, starting the process all over again.
5 0;the result of the addition is written at memory square 5. Memory square 5 initially has a value of 0 in it.
A program to multiply two numbers together.
;*************************
;A program to multiply 7*5
;this program writes the result of mulitplying 7 times 5 to memory square 31. It does this by
;repeatedly adding the number 7, found at memory address 30, to memory square 31. After 5 such additions, the program stops.
;*************************
1 CLA ;clear the accumulator
2 ADD 31 ;memory square 31 is where the result of the multiplication will be stored.
3 ADD 30 ;add the number being multiplied to the accumulator. Because 7 is being multiplied by 5, the 7 found at memory square 30 is added to the accumulator
4 STA 31 ;write the new current "product" back into memory
5 CLA ;clear accumulator, get ready for new arithmetic operation
6 ADD 29 ;put current result of subtraction into accumulator
7 SUB 28 ;Decrement counter by 1
8 STA 29 ;save current value of counter
9 JZ 27 ;stop the program once the result of the subtraction equals 0
10 JMP 1 ;jump back to 1
27 STP ;stop the program
28 1 ;amount to decrement counter by
29 4 ;counter
30 7 ;amount to multiply by
31 0 ;store product here
It might not seem at this point that you can do very much with these 8 instructions and 6 rules. After all, the computer programs listed above only perform menial arithmetic operations. By comparison, modern computers and software can do everything from automating factories to surfing the internet.
However, an amazing fact about computers is that what one programmable computer can do, any programmable
computer can do given enough time and memory. The person who made this discovery is Alan Turing, who was one
of the primary inventors of the modern day digital computer. The idea is called the theory of the Universal Computer.
|